FBMC
Filter Bank Modulation Carrier
Technology behind FBMC
Filter Bank Multicarrier (FBMC) systems are like OFDM a subclass of multicarrier systems.FBMC can be seen as an envolved form of OFDM which overcomes the limitations of OFDM (loss in spectral efficiency due to cyclic prefix insertion) by adding generalized pulse shaping filters which delivers a well localized subchannel in both time and frequency domain. Consequently, FBMC systems have more spectral containment signals and offer more effective use of the radio resources where no cyclic prefix is required.
OFDM: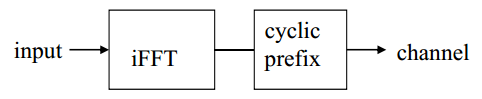
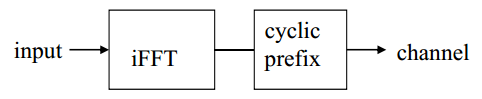
FBMC: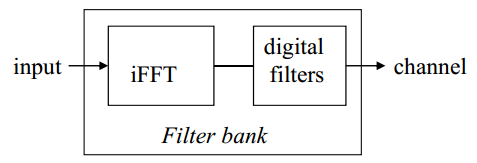
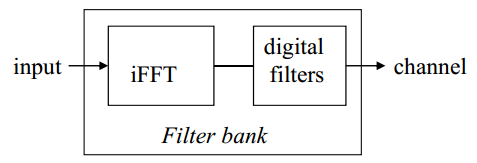
Implementation approach
Like in OFDM the Transmitted Data is split up onto many small carriers. The idea is, to use the same carrier allocation as described in OFDM and link the signal for each carrier to wilson bases instead of using a cyclic prefix. To handle and transmit the real and the complex part of a symbol a bit padder is needed.Diagram: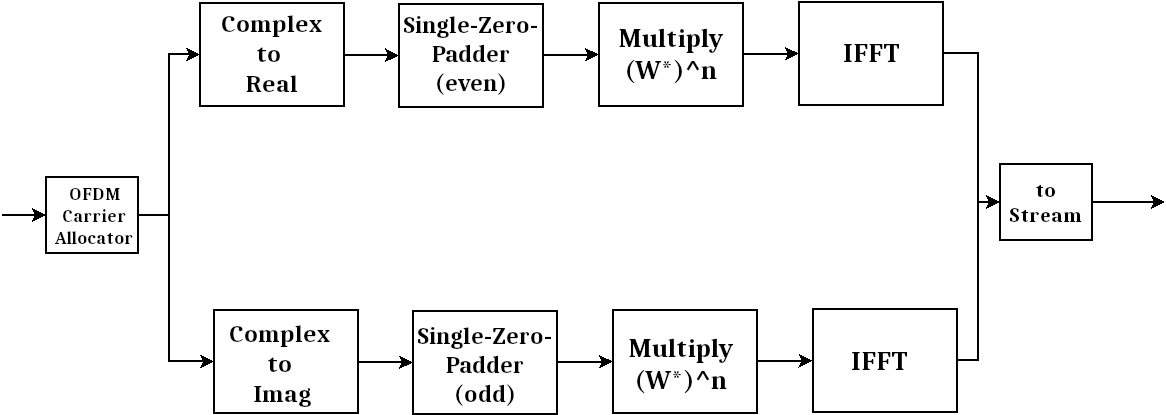
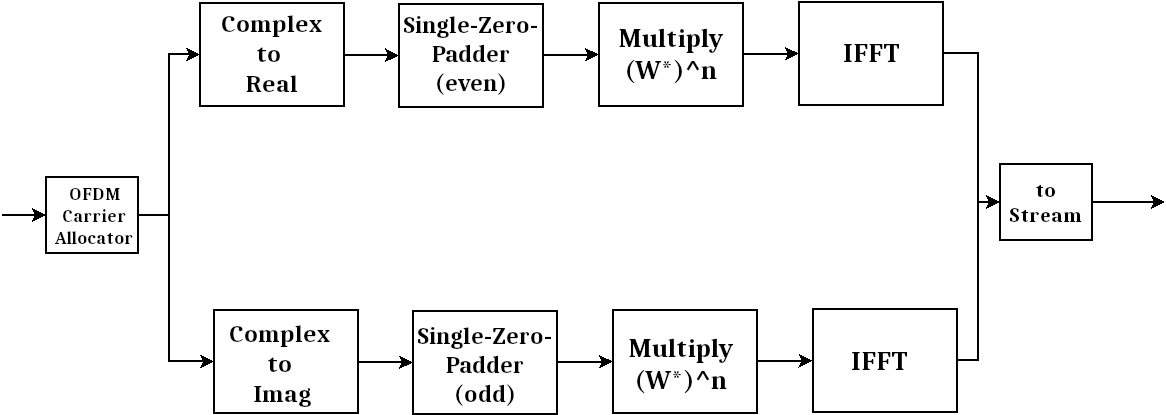
Spectrum: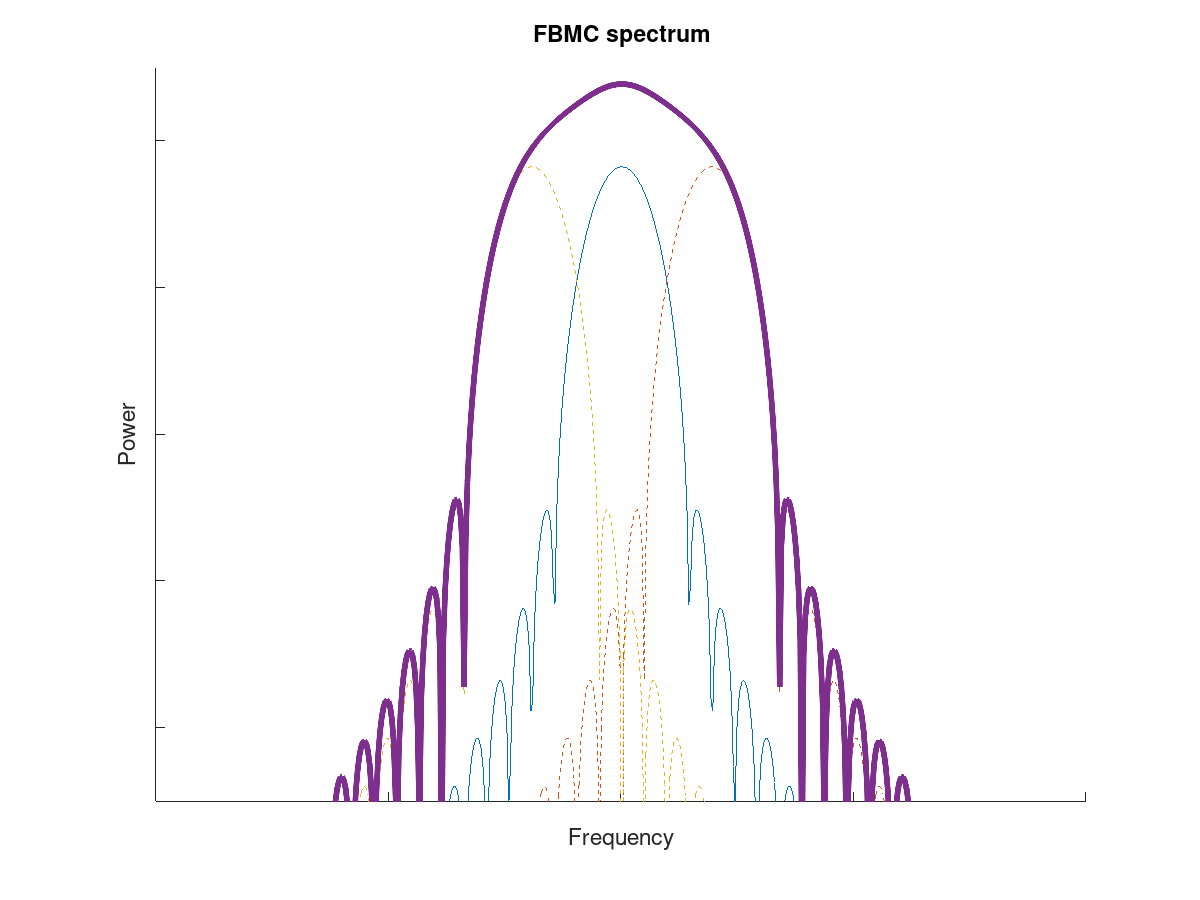
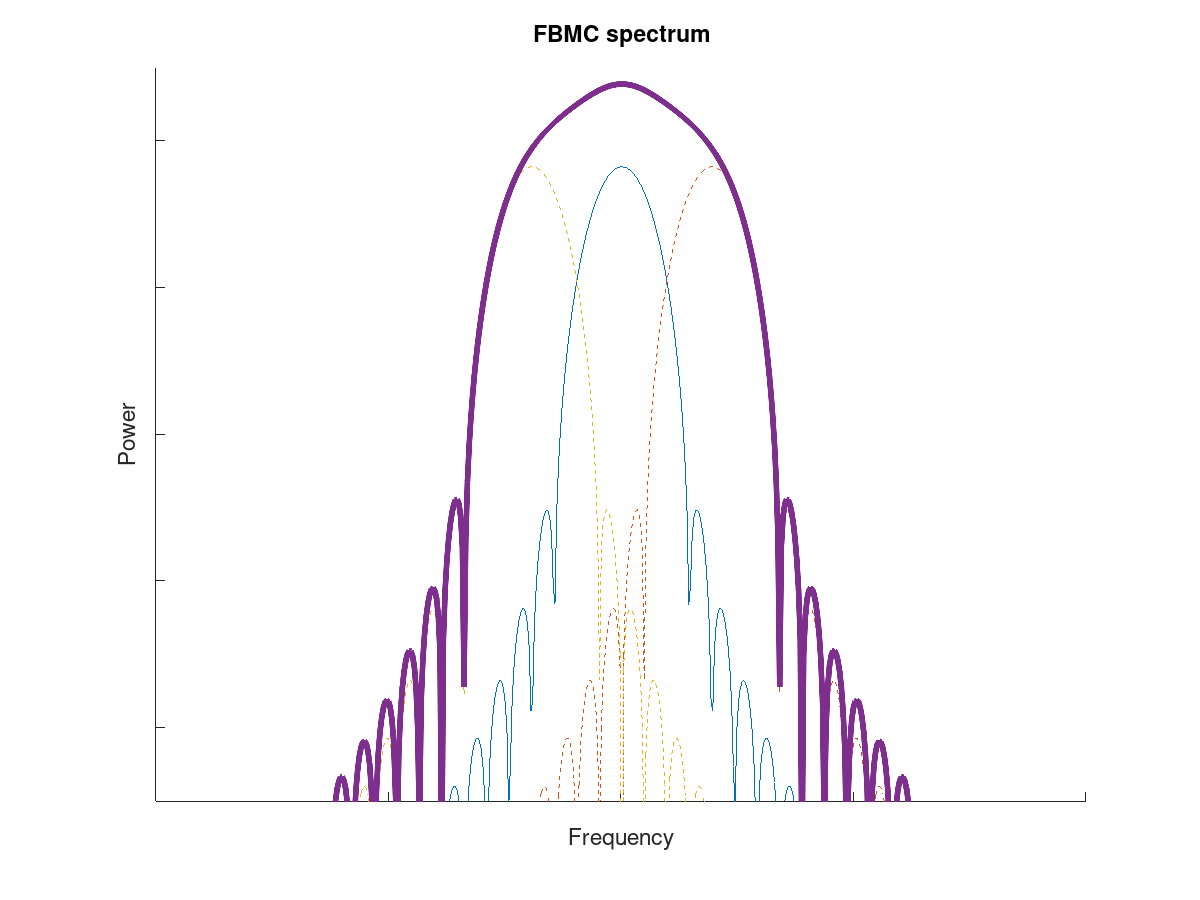
Gnuradio Block
A bit padding block like described above does not exist in Gnuradio. In this section its is shown how to this type of block was created.Diagram: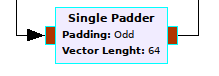
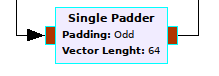
Out Of Tree Module
At first an Gnuradio Out Of Tree Module had to be created using a commandline program named gr_modtool. This creates an basic skeleton source code and directory structure.$ gr_modtool newmod fbmc
Creating out-of-tree module in ./gr-howto... Done.
$ gr_modtool add -t general -l cpp single_padder
GNU Radio module name identified: howto
Block/code identifier: square_ff
Language: C++
C++ Source
In the source code the zero padding bits were added, depending on the even/odd boolean.Block Constructor
Twice the amount of input data needs to be allocated in the block constructor.
single_padder_impl::single_padder_impl(bool even, int vec_len)
: gr::sync_block("single_padder",
gr::io_signature::make(1, 1, vec_len * sizeof(float)),
gr::io_signature::make(1, 1, vec_len * 2 * sizeof(float))) // double output lenght
{
m_Even = even;
m_VectLen = vec_len;
}
Work Function
This is the work function which does the calculation. The work function is called by the Gnuradio scheduler. int
single_padder_impl::work(int noutput_items,
gr_vector_const_void_star &input_items,
gr_vector_void_star &output_items)
{
const float *in = (const float *) input_items[0];
float *out = (float *) output_items[0];
for(int i = 0; i < noutput_items * m_VectLen; i++)
{
out[i * 2 + (int)(m_Even)] = in[i];
out[i * 2 + (int)(!m_Even)] = 0.0;
}
// Tell runtime system how many output items we produced.
return noutput_items;
}
GRC Description
In the last step a Gnuradio Companion description file had to be created. This file defines the ports and configuration options avaiable within the graphical Gnuradio Companion editor.<block>
<name>Single Padder</name>
<key>fbmc_single_padder</key>
<category>[FBMC]</category>
<import>import fbmc</import>
<make>fbmc.single_padder($even, $vec_len)</make>
<param>
<name>Padding</name>
<key>even</key>
<type>bool</type>
<option>
<name>Even</name>
<key>True</key>
</option>
<option>
<name>Odd</name>
<key>False</key>
</option>
</param>
<param>
<name>Vector Lenght</name>
<key>vec_len</key>
<type>int</type>
</param>
<sink>
<name>in</name>
<type>float</type>
<vlen>$vec_len</vlen>
</sink>
<source>
<name>out</name>
<type>float</type>
<vlen>2* $vec_len</vlen>
</source>
</block>
Build and Install
The Gnuradio build system uses CMake, so following commands need to be used within the module folder to build and install the block:
$ mkdir build
$ cd build/
$ cmake ..
$ make -j8
$ sudo make install